Puede crear una amplia gama de formas, efectos y declaraciones de texto utilizando Scripts de Forma, para mejorar la apariencia y valor de información de los elementos y conectores que crea. Algunos ejemplos de tales scripts se proporcionan aquí.
Acceso Configuraciones | Tipos de UML > Estereotipos (especificar estereotipo): Script de Forma + Asignar o
Configuraciones | Tipos de UML > Estereotipos (especificar estereotipo): Script de Forma + Editar
Ejemplos de Script de Forma
Script
|
Forma
|
// BASIC SHAPES
shape main
{
setfillcolor(255,0,0); // (R,G,B)
rectangle(0,0,90,30); // (x1,y1,x2,y2)
setfillcolor(0,255,0); // (R,G,B)
ellipse(0,30,90,60); // (x1,y1,x2,y2)
setfillcolor(0,0,255); // (R,G,B)
rectangle(0,60,90,90); // (x1,y1,x2,y2)
}
|
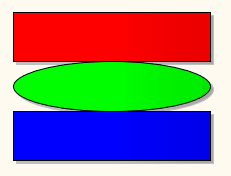
|
// SINGLE CONDITIONAL SHAPE
shape main
{
if (HasTag("Trigger","Link"))
{// Sólo dibujar si el objeto tiene un Valor Etiquetado
// Disparador=Vínculo
// Establecer el color de relleno para la ruta
setfillcolor(0,0,0);
startpath(); // Empezar a trazar una ruta
moveto(23,40);
lineto(23,60);
lineto(50,60);
lineto(50,76);
lineto(76,50);
lineto(50,23);
lineto(50,40);
endpath(); // Terminar de trazar una ruta
// Llenar la ruta de traza con el color de relleno
fillandstrokepath();
return;
}
}
|
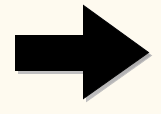
|
// MULTI CONDITIONAL SHAPE
shape main
{
startpath();
ellipse(0,0,100,100);
endpath();
fillandstrokepath();
ellipse(3,3,97,97);
if (HasTag("Trigger","None"))
{
return;
}
if (HasTag("Trigger","Error"))
{
setfillcolor(0,0,0);
startpath();
moveto(23,77);
lineto(37,40);
lineto(60,47);
lineto(77,23);
lineto(63,60);
lineto(40,53);
lineto(23,77);
endpath();
fillandstrokepath();
return;
}
if (HasTag("Trigger","Message"))
{
rectangle(22,22,78,78);
moveto(22,22);
lineto(50,50);
lineto(78,22);
return;
}
}
|
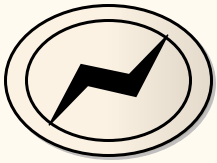
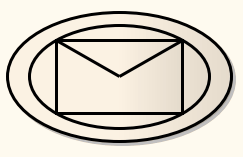
|
// SUB SHAPES
shape main
{
rectangle(0,0,100,100);
addsubshape("red", 10, 20);
addsubshape("blue", 30, 40);
addsubshape("green", 50, 20);
addsubshape("red", 100, 20);
shape red
{
setfillcolor(200, 50, 100);
rectangle(0,0,100,100);
}
shape blue
{
setfillcolor(100, 50, 200);
rectangle(0,0,100,100);
}
shape green
{
setfillcolor(50, 200, 100);
rectangle(0,0,100,100);
}
}
|
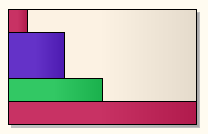
|
// EDITABLE FIELD SHAPE
shape main
{
rectangle(0,0,100,100);
addsubshape("namecompartment", 100, 20);
addsubshape("stereotypecompartment", 100, 40);
shape namecompartment
{
h_align = "center";
editablefield = "name";
rectangle(0,0,100,100);
println("name: #name#");
}
shape stereotypecompartment
{
h_align = "center";
editablefield = "stereotype";
rectangle(0,0,100,100);
println("stereotype: #stereotype#");
}
}
|
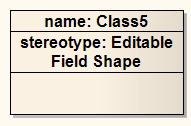
|
// RETURN STATEMENT SHAPE
shape main
{
if(hasTag("alternatenotation", "false"))
{
//dibujar glifo incorporado del ea
drawnativeshape();
//salir del script con la declaración de devolución
return;
}
else
{
//alternar comandos de notación
//...
rectangle(0,0,100,100);
}
}
|
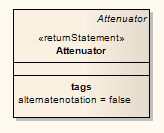
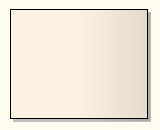
|
//EMBEDDED ELEMENT SHAPE POSITION ON PARENT EDGE
shape main
{
defsize(60,60);
startpath();
if(hasproperty("parentedge","top"))
{
moveto(0,100);
lineto(50,0);
lineto(100.100);
}
if(hasproperty("parentedge","bottom"))
{
moveto(0,0);
lineto(50.100);
lineto(100,0);
}
if(hasproperty("parentedge","left"))
{
moveto(100,0);
lineto(0,50);
lineto(100.100);
}
if(hasproperty("parentedge","right"))
{
moveto(0,0);
lineto(100,50);
lineto(0.100);
}
endpath();
setfillcolor(153,204,255);
fillandstrokepath();
}
|
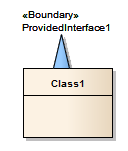
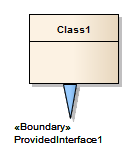 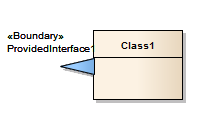 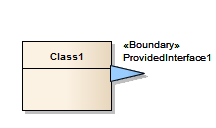
|
// CLOUD PATH EXAMPLE SHAPE
shape main
{
StartCloudPath();
Rectangle(0,0,100,100);
EndPath();
FillAndStrokePath();
}
|
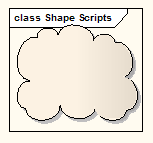
|
// CONNECTOR SHAPE
shape main
{
// dibujar una línea punteada
noshadow=true;
setlinestyle("DASH");
moveto(0,0);
lineto(100,0);
}
shape source
{
// dibujar un círculo en el extremo fuente
rotatable = true;
startpath();
ellipse(0,6,12,-6);
endpath();
fillandstrokepath();
}
shape target
{
// dibujar una cabeza de flecha en el extremo destino
rotatable = true;
startpath();
moveto(0,0);
lineto(16,6);
lineto(16,-6);
endpath();
fillandstrokepath();
}
|
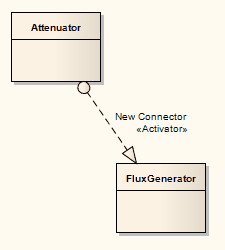
|
// DOUBLE LINE
shape main
{
setlinestyle("DOUBLE");
moveto(0,0);
lineto(100,0);
}
|
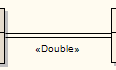
|
// ROTATION DIRECTION
shape main
{
moveto(0,0);
lineto(100,0);
setfixedregion(40,-10,60,10);
rectangle(40,-10,60,10);
if(hasproperty("rotationdirection","up"))
{
moveto(60,-10);
lineto(50,0);
lineto(60,10);
}
if(hasproperty("rotationdirection","down"))
{
moveto(40,-10);
lineto(50,0);
lineto(40,10);
}
if(hasproperty("rotationdirection","left"))
{
moveto(40,-10);
lineto(50,0);
lineto(60,-10);
}
if(hasproperty("rotationdirection","right"))
{
moveto(40,10);
lineto(50,0);
lineto(60,10);
}
}
|

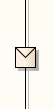
|
// GET A VALUE RETURNED BY AN ADD-IN
shape main
{
//Dibujar un simple rectángulo
Rectangle(0,0,100,100);
//Imprimir valor de cadena devuelto desde el Add-In "MyAddin",
//Función "MyExample" con dos parámetros de cadena
Print("#ADDIN:MyAddin, MyExample, param1, param2#");
}
// METHOD SIGNATURE FOR ADD-IN FUNCTION:
// Función Pública MyExample(Repository As EA.Repository,
// eaGuid As String, args As Variant) As Variant
|
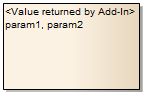
|
// ADD CUSTOM COMPARTMENTS BASED UPON CHILD ELEMENTS
// OR RELATED ELEMENTS
( Ver Agregar Compartimientos Personalizados a Elemento )
|
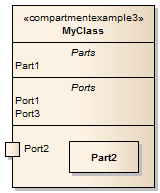
|
// RETURN THE INCOMING AND OUTGOING EDGE FOR CONNECTORS
// GOING INTO AND OUT OF AN OBJECT
shape main
{
//Dibujar un simple rectángulo
Rectangle(0,0,100,100);
//Imprimir extremos entrantes en el elemento
Print("Incoming Edge: #incomingedge#\n");
//Imprimir extremos salientes en el elemento
Print("Outgoing Edge: #outgoingedge#\n");
}
|
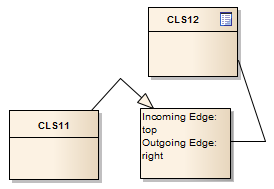
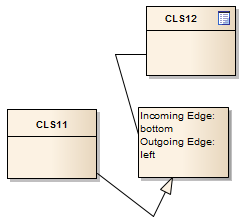
|
// CHECK WHETHER A COMPOSITE ELEMENT ICON IS REQUIRED AND,
// IF SO, DRAW ONE
decoration comp
{
orientation="SE";
if(hasproperty("IsDrawCompositeLinkIcon","true"))
{
startpath();
ellipse(-80,29,-10,71);
ellipse(10,29,80,71);
moveto(-10,50);
lineto(10,50);
endpath();
strokepath();
}
}
|
|
Aprender más
Temas de Centro de aprendizaje
•
|
(Alt+F1) | Enterprise Architect | Lenguajes de Modelado | Definiendo Formas | Definiendo la Forma de un Elemento
|
•
|
(Alt+F1) | Enterprise Architect | Lenguajes de Modelado | Definiendo Formas | Definiendo la Forma de un Conector
|
|